Posts
Showing posts from 2018
CORS support in Web API
- Get link
- Other Apps
If you read the Web API tutorials from docs.microsoft.com, all of them are teaching you to create the server app (Web API) and the client app in the same solution. In fact, we usually separate the server and client application into separate applications. You will then find out the client application cannot call any Web API method in the server application. It is because of the CORS. What is CORS? CORS stands for Cross-Origin Resource Sharing. CORS is a W3C standard that allows a server to relax the same-origin policy. Using CORS, a server can explicitly allow some cross-origin requests while rejecting others. CORS is safer and more flexible than earlier techniques such as JSONP . This tutorial shows how to enable CORS in your Web API application. If your application is ASP.NET Web API 2, now you could do the following to enable CORS Install Microsoft.AspNet.WebApi.Cors from Nuget Open file App_Start/WebApiConfig.cs. In Register method, add this code config.EnableCors(); You can
Dependency injection in C#
- Get link
- Other Apps
What is Dependency Injection? Dependency Injection (DI) is a software design pattern. It allows us to develop loosely-coupled code. How does it do that? DI reduces the hard-coded dependencies among your classes by injecting those dependencies at runtime/compile time instead of design time technically. Thus it reduces the tight coupling among different software components. It also helps in improving the code readability and testability. Now we know that there quite a lot of advantages using Dependency injection, lets go ahead and identify how many different kinds of Dependency injections are there and how do we go about implementing them. Constructor Injection This is most commonly used dependency injection pattern in object oriented programming. The constructor injection normally has only one parameterized constructor, so in this constructor dependency there is no default constructor and we need to pass the specified value at the time of object creation. We can use the injection
Which messaging queue is best
- Get link
- Other Apps
Messaging is one of the most important aspects of modern programming techniques. Majority of today’s systems consist of several modules and external dependencies. If they weren’t able to communicate with each other in an efficient manner, they wouldn’t be very effective in carrying out their intended functions. There are quite a few messaging queues available on the cloud today, some of the popular ones being Apache Kafka, RabbitMQ, Amazon SQS, Google Cloud Pub/Sub etc. There is no doubt that we need a messaging queue for several modules but which one? In this blog post, I would like to give a try on answering that question. RabbitMQ RabbitMQ is an open source message broker software managed by Pivotal. It implements the Advanced Message Queuing Protocol (AMQP). RabbitMQ comes with a built-in management dashboard for easy configuration and monitoring of message queues. It also supports requests for message acknowledgments and is easily deployed in HA mode with minimal effort. With a
How to check what data of yours Google has gathered
- Get link
- Other Apps
Here is how you can check what data of yours has Google gathered: https://www.google.com/maps/timeline?pb Google stores your location (if you have it turned on) every time you turn on your phone, and you can see a timeline from the first day you started using Google on your phone. . https:// myactivity.google.com/myactivity Google stores search history across all your devices on a separate database, so even if you delete your search history and phone history, Google STILL stores everything until you go in and delete everything, and you have to do this on all devices http://www. google.com/settings/ads/ Google creates an advertisement profile based on your information, including your location, gender, age, hobbies, career, interests, relationship status, possible weight (need to lose 10lbs in one day?) and income https://myaccount.google.com/permissions Google stores information on every app and extension you use, how often you use them, where you use them, and wh
How to check what data of yours has Facebook gathered
- Get link
- Other Apps
Facebook in past few weeks has seen the biggest onslaught both from media and from users. Facebook is accused of sharing the data of users to UK based consulting firm, Cambridge Analytica. Now if you want to see what data of yours is being gathered and shared with Ad Agencies and other firms, here is what you should do: From your desktop, visit the page – https://register.facebook.com/download/ Clicking on the link will lead you to your account setting page. Below the General Account Settings option click on ‘Download a copy’ link. Once clicked, you will see a ‘Download your information’ page with an option to click on ‘Download Archive’. Clicking on that will open a dialogue box asking for your password. Once the password is provided, there will be a prompt saying that Facebook will notify you once the data is ready to download. Once ready, you can click on the notification and download the .zip file on the desktop. After downloading, extract the files and click on ‘HTML’
Introduction to OData
- Get link
- Other Apps
OData (Open Data Protocol) is an open protocol for sharing the data. It is OASIS (Organization for the Advancement of Structured Information Standards) standard which defines best practice for building and consuming REST API. The main goal of the Open Data protocol is to any application can able to access from any other application. Nowadays many applications like Web browsers, apps on mobile devices, BI tools are required to access common data sources. The data source of every application has its own approach and style so it is very difficult to create the data source that can be accessed by all applications. Solution to this problem is to define common approaches and that all application owners agree to follow this approach for accessing the data. Currently, OData is widely used for exposing data. The applications such as Facebook and eBay are exposing their data via OData. OData is preferred to use custom enterprise applications for expose data. To do this OData libraries are a
Create a Central Logging System
- Get link
- Other Apps
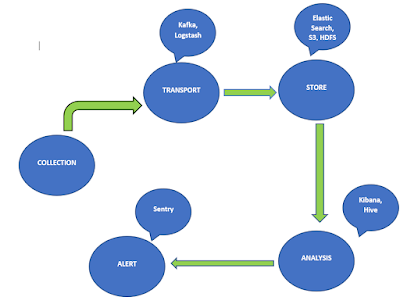
Logging is the most important part of any system. They give you insights about the application, what kind of errors are occurring and what components are causing the errors and also about how the application is reacting and working when something wrong happens. The usual practice is almost every application write to logs either in the file system or in Database. If your application is running on multiple hosts then designing a central logging system becomes crucial since you can collect, aggregate and maintain logs at one centralized location and do operations on top of them. There are many tools available to which can solve some part of the problem but we need to build a robust application using all these tools. Create a Centralised Logging Application Collection Imagine you have a web application running on the server and if something goes down, your developers or operations team need to access lo
Introduction to API Streaming
- Get link
- Other Apps
API Streaming Introduction let’s say that you want to have a look at stock market prices. You launch your app on your phone. The app uses APIs to retrieve data, in our case stock market prices, and display it on the screen. Ten seconds later, those prices have probably changed. You don’t know, but chances are at least some of them have changed. So, you’re going to hit the refresh button to reload. By reloading the page, you’re going to ask again through the same API to get the new prices. With API Streaming you would no longer have to hit the refresh button. You would receive new prices as soon as they are available, the interface would be updated in real-time with no effort required from you, and no frustration to be late on the market. Value Prop? For time-sensitive data, the way APIs work today makes them consume and waste a lot of resources without being accurate in all situations. With API Streaming, we would be able to deliver accurate and fresh data from APIs while redu
Introduction to API Gateway
- Get link
- Other Apps
Introduction to API Gateway What is API Gateway API Gateway can be considered a backplane to connect and other public or private websites. It provides RESTful application programming interfaces (APIs) for mobile and web applications to access its services. Its a one-stop shop for the client, anything client wants API Gateway will get it for him. Problem How do the clients of a Microservices-based application access the individual services? Challenges The number of service instances and their locations changes dynamically Services might use a diverse set of protocols, some of which might not be web friendly Different clients need different data. For example, the desktop browser version of a product details page desktop is typically more elaborate than the mobile version. Partitioning into services can change over time and should be hidden from clients Solution Implement an API gateway that is the single entry point for all clients. The API gateway handles requests
Introduction to Service Workers
- Get link
- Other Apps
Introduction to service workers Service Workers are the core components of Progressive Web Apps (PWA), because they allow caching of resources, which will help in offline experience and push notifications, which help for re marketing and engagement, two of the main distinguishing features that up to now set native apps apart. Introduction to Service workers A Service Worker is essentially programmable proxy between web page and the network, it gives ability to intercept and cache network requests, effectively giving the ability to create an offline experience for web app, which is one of the biggest advantages of native app over web app. It’s a special kind of web worker, a JavaScript file associated with a web page which runs on a worker context, separate from the main thread, giving the benefit of being non-blocking — so computations can be done without sacrificing the UI responsiveness. Being on a separate thread it has no DOM access, and no access to the Local Storage
Introduction to Git
- Get link
- Other Apps
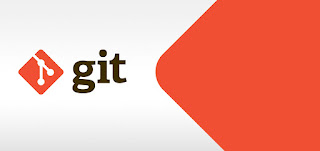
Git is a free and Open Source version control system, a technology used to track older versions of files, providing the ability to roll back and maintain separate different versions at the same time. Git is a free ware and is a successor of SVN and CVS, two very popular version control systems of the past. First developed by Linus Torvalds (the creator of Linux), today is the go-to system which you can’t avoid if you make use of Open Source software. Introduction to Git VCS How does it work? Git is a distributed system. Many developers can clone a repository from a central location, work independently on some portion of code, and then commit the changes back to the central location where everybody updates. Git makes it very easy for developers to collaborate on a codebase simultaneously and provides tools they can use to combine all the independent changes they make. A very popular service that hosts Git repositories is GitHub, especially for Open Source software, bu
Is Go an Object Oriented Language?
- Get link
- Other Apps

Sometimes I read an article that says “Go is object oriented”. Sometimes another article that claims that no object oriented programming can be done with Go, just because it does not have classes. So I wrote this post to clarify this topic. Is Go object oriented, or not? Yes , Go is object oriented. Here is what GO FAQ says: Yes and no. Although Go has types and methods and allows an object-oriented style of programming, there is no type hierarchy. The concept of “interface” in Go provides a different approach that we believe is easy to use and in some ways more general. There are also ways to embed types in other types to provide something analogous—but not identical—to subclassing. Moreover, methods in Go are more general than in C++ or Java: they can be defined for any sort of data, even built-in types such as plain, “unboxed” integers. They are not restricted to structs (classes). Also, the lack of a type hierarchy makes “objects” in Go feel much more lightweight than i
Push API Tutorial
- Get link
- Other Apps

The Push API allows a web app to receive messages pushed by a server, even if the web app is not currently open in the browser or not running on the device. What is Push API? The Push API is a recent addition to the browser APIs, and it’s currently supported by Chrome (Desktop and Mobile), Firefox and Opera since 2016. Microsoft Edge supports it in Preview version and Safari already has its own implementation. Why should we use Push API? You can send messages to your users, pushing them from the server to the client, even when the user is not browsing the site. This lets you deliver notifications and content updates, hence better customer engagement. This was one of the missing components of mobile web when compared to Native apps and along with this, offline support as well. How does it work? When a user visits your web app, you can trigger a panel asking permission to send updates or receive offers or notifications. A Service Worker is installed, and operating in t